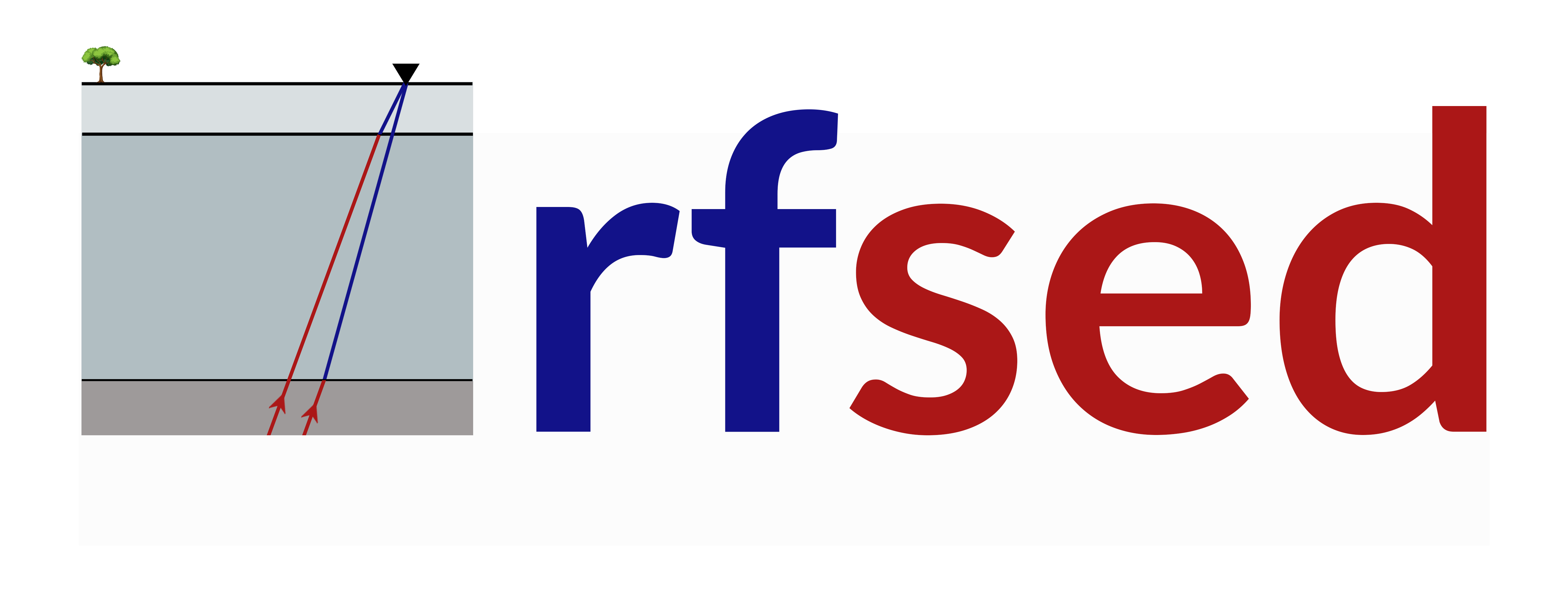
Module synrf
Class for calculating synthetic receiver function using the forward modelling method.
Example
>>> # Initialize the synrf module:
>>> from rfsed.synrf import synrf
>>> # Define the Earth model parameters
>>> import numpy as np
>>> depth = np.array([2, 35, 77.5])
>>> vp = np.array([2.0, 6.5, 8.045])
>>> vs = np.array([1.36, 3.75, 4.485])
>>> rho=np.array([2.72, 2.92, 3.3455])
>>> preonset=5
>>> n=2100
>>> rayp=0.04
>>> gaussian=1.25
>>> delta=0.025
>>> # Call the synrf class
>>> Synth=synrf(depth, vp, vs, rho, rayp, dt=delta, npts=n, ipha=1)
>>> Synth.run_fwd()
>>> Synth.filter(freqmin=0.05, freqmax=1.25, order=2, zerophase=True)
>>> rf_synth=Synth.run_deconvolution(pre_filt=[0.05, 1.25],
preonset=preonset, gaussian=gaussian)
>>> trdata=(rf_synth[0]).data