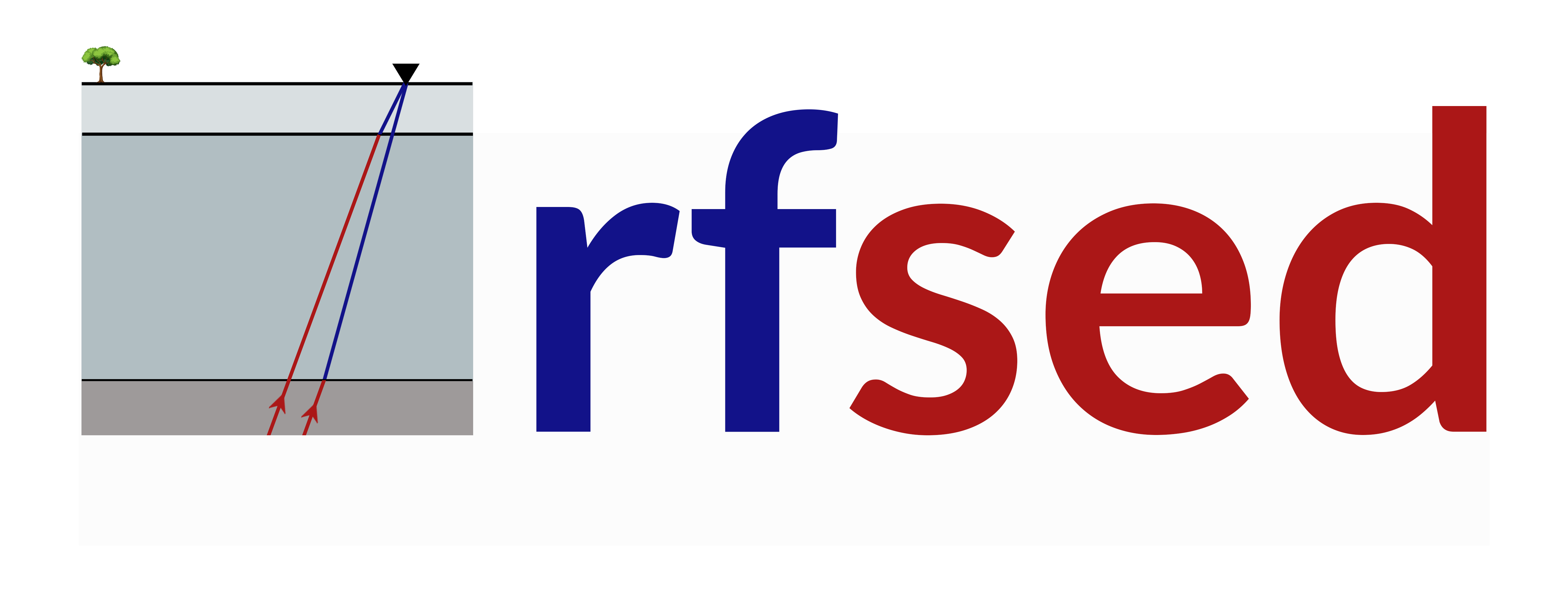
Module WaveformFitting
- rfsed.WaveformFitting.WaveformFitting(rfstream, preonset, HSed, VpSed, VpCrust, rayp, KMoho, HMoho, gaussian, wtCorr, wtRMSE, wtPG, savepath, format)
This function performs waveform fitting for the Moho depth and Vp/Vs ratio Returns the best model based on the Correlation Coefficient (CC), Root-mean-squared error (RMSE), Phase-goodness-of-fit (PG) and Overall Goodness-of-fit that combines CC, RMSE, and PG. Weights of CC, RMSE and PG (wtCorr,wtRMSE, wtPG) are given as input and can be modified before plotting. At all times, (wtCorr + wtRMSE + wtPG) = 1.0
- Parameters:
rfstream (RFStream) – RFStream object containing the receiver functions
preonset (integer) – time in seconds before the P-arrival
HSed (float) – Sediment thickness in km
VpSed – Sediment Vp in km/s
VpSed – float
VpCrust – Crustal Vp in km/s
VpCrust – float
rayp – Ray parameter in s/km
rayp – float
KMoho (numpy array) – Vp/Vs ratio range for Moho
HMoho (numpy array) – Moho depth range in km
gaussian (float) – Gaussian filter parameter (a)
wtCorr (float) – Weight of Correlation Coefficient
wtRMSE (float) – Weight of Root-mean-squared error
wtPG (float) – Weight of Phase Goodness of Fit
savepath (str) – Path to save the plots
format (str) – Format of the plots
- Returns:
Dictionary containing the Waveform Fitting Results
Example
>>> # Initialise the Waveform Fitting module >>> from rfsed.WaveformFitting import WaveformFitting >>> # Define all the necessary parameters >>> import numpy as np >>> # rfstream is a RFStream object containing the receiver functions (based on rf) >>> rfstream = rfstream >>> preonset = 10 >>> HSed = 1.5 >>> VpSed = 2.5 >>> VpCrust = 6.5 >>> rayp = 0.06 >>> KMoho = np.arange(1.6, 1.9, 0.01) >>> HMoho = np.arange(20, 50, 201) >>> gaussian = 1.25 >>> wtCorr, wtRMSE, wtPG = [0.4, 0.4, 0.2] >>> savepath = 'path/to/saveplots' >>> format = 'png' >>> # Call the WaveformFitting function >>> WaveformFitting(rfstream, preonset, HSed, VpSed, VpCrust, rayp, KMoho, HMoho, gaussian, wtCorr, wtRMSE, wtPG, savepath, format)
- rfsed.WaveformFitting.PlotWaveformFitting(WaveformFitting, wtCorr, wtRMSE, wtPG, savepath, format)
This function plots the waveform fitting results for the Moho depth and Vp/Vs ratio. Plots the Waveform Fitting Results for CC, RMSE, PG and GoF. Weights of CC, RMSE and PG are given as input and can be modified before plotting. At all times, wtCorr + wtRMSE + wtPG = 1.0
- Parameters:
WaveformFitting (dict) – Dictionary containing the Waveform Fitting Results from the function WaveformFitting
wtCorr (float) – Weight of Correlation Coefficient
wtRMSE (float) – Weight of Root-mean-squared error
wtPG (float) – Weight of Phase Goodness of Fit
savepath (str) – Path to save the plots
format (str) – Format of the plots
- Returns:
Plots the Waveform Fitting Results for CC, RMSE, PG and GoF
Example
>>> # Initialise the PlotWaveformFitting module >>> from rfsed.WaveformFitting import PlotWaveformFitting >>> # Define all the necessary parameters >>> # WaveformFitting parameter is an output from the WaveformFitting function, >>> # a dictionary containing the Waveform Fitting Results >>> # (see WaveformFitting function for details) >>> WaveformFitting = WaveformFitting(rfstream, preonset, HSed, VpSed, VpCrust, rayp, KMoho, HMoho, gaussian, wtCorr, wtRMSE, wtPG, savepath, format) >>> wtCorr, wtRMSE, wtPG = [0.4, 0.4, 0.2] >>> savepath = 'path/to/saveplots' >>> format = 'png' >>> # Call the PlotWaveformFitting function >>> PlotWaveformFitting(WaveformFitting, wtCorr, wtRMSE, wtPG, savepath, format)