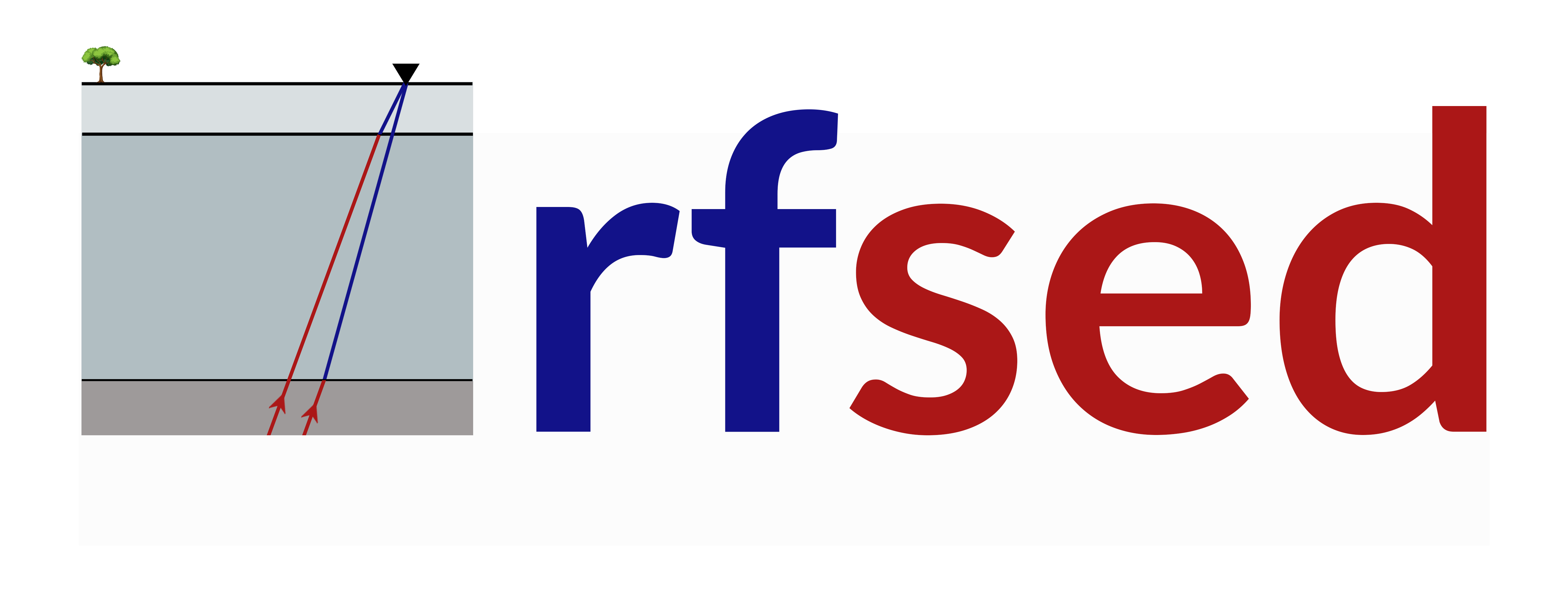
Module hkZhu
- rfsed.hkZhu.getamp(rfdata, tarray, t)
Get the amplitude of the receiver function at a specific time
- Parameters:
rfdata – receiver function data
tarray – time array
t – time to get the amplitude
- Returns:
Amplitude of the receiver function at time t
- rfsed.hkZhu.hk(rfstream, preonset, H, K, Vp, w1, w2, w3, layer, stack=False)
Calculate the H-K stacking for a receiver function after Zhu and Kanamori (2000)
- Parameters:
rfstream (RFStream) – receiver function stream
preonset (integer) – time in seconds before the P-arrival
H (numpy array) – array of Depth values
K (numpy array) – array of Vp/Vs values
Vp (float) – P wave velocity of the layer
w1 – weight for the Ps arrival
w2 – weight for the PpPs arrival
w3 – weight for the PsPs+PpSs arrival
layer (str) – layer name (‘Moho’ or ‘Sed’)
stack (bool) – stack the receiver functions before the H-K stacking (default False)
- Returns:
Dictionary of H-K stacking results
Example
>>> # Initialize the hk module: >>> from rfsed.hkZhu import hk >>> # Define input data and all the necessary parameters. The input data should >>> # be in the form of RFStream object from rf package >>> import numpy as np >>> from rf.rfstream import read_rf >>> rfstream = read_rf('path/to/rfdata') >>> preonset = 10 >>> H = np.linspace(20,60,201) >>> K = np.linspace(1.65,1.95,121) >>> Vp = 6.9 >>> w1, w2, w3 =[0.6, 0.3, 0.1] >>> layer = 'Moho' >>> stack = False >>> # Call the hk function >>> hk(rfstream, preonset, H, K, Vp, w1, w2, w3, layer, stack)
- rfsed.hkZhu.plothk(HKResult, savepath, g=[75.0, 10.0, 15.0, 2.5], rmneg=True, format='jpg')
Plot the H-K stacking results
- Parameters:
HKResult (dict) – Dictionary of H-K stacking results from hk function
savepath (str) – path to save the figure
g (list) – list of gain values for the plot
rmneg (bool) – remove the negative values from the stack (default True)
format (str) – format of the figure
- Returns:
Plot of the results from the Sequential H-K stacking method
Example
>>> # Initialize the hk plotting module: >>> from rfsed.hkZhu import plothk >>> # Define input data (which is the result from the hk function) >>> # and other plotting parameters. >>> HKResult=hk(rfstream, preonset, H, K, Vp, w1, w2, w3, layer, stack) >>> savepath = 'path/to/save/figure' >>> g = [75.,10., 15., 2.5] >>> rmneg = True >>> format = 'pdf' >>> # Call the plothk function >>> plothk(HKResult, savepath, g, rmneg, format)