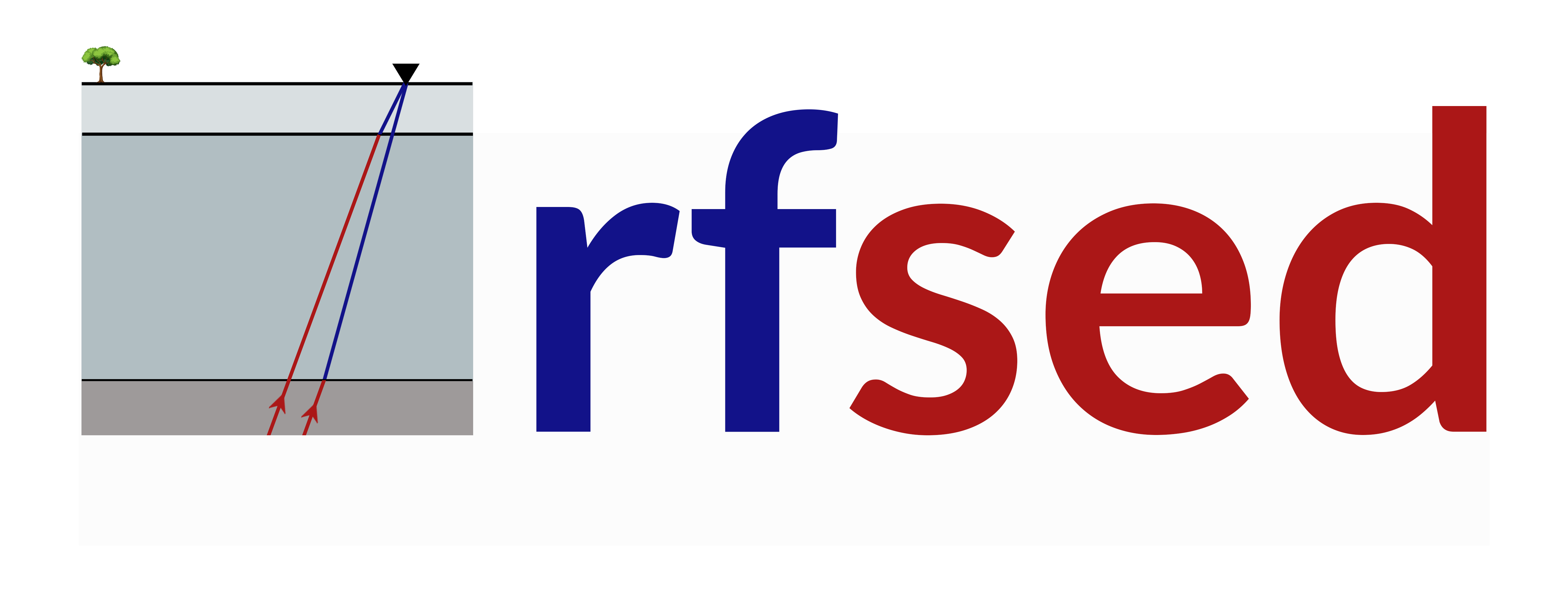
Module ReverbFilter
- rfsed.ReverbFilter.getamp(rfdata, tarray, t)
Get the amplitude of the receiver function at a specific time
- Parameters:
rfdata (numpy array) – receiver function data
tarray (numpy array) – time array
t (float) – time to get the amplitude
- Returns:
Amplitude of the receiver function at time t
- rfsed.ReverbFilter.ResonanceFilt(rfstream, preonset)
Resonance Filter for receiver function data (Removes sediment reverberation signal)
- Parameters:
rfstream (obspy stream) – receiver function data
preonset (integer) – time in seconds before the P-arrival
- Returns:
A dictionary of stacked receiver function, Filtered receiver function, autocorrelation of the data, resonance filter, time lag (2 way travel time of the sediment reverbration), and the strength of the sediment reverbration (r)
Example
>>> # Initialize the ResonanceFilt module: >>> from rfsed.ReverbFilter import ResonanceFilt >>> # Define all the necessary parameters >>> # The rfstream data is a RFSream object from rf >>> from rf.rfstream import read_rf >>> rfstream = read_rf('path/to/rfdata') >>> preonset = 10 >>> # Call the ResonanceFilt function >>> ResonanceFilt(rfstream, preonset)
- rfsed.ReverbFilter.plotfiltrf(FilteredRF, savepath, format='jpg')
Plot the filtered receiver function, autocorrelation, and the resonance filter
- Parameters:
FilteredRF (dict) – Dictionary of results from the resonance filtering method (function: Resonance_Filt)
savepath (str) – path to save the plots
format (str) – format of the plot (default: jpg)
- Returns:
Plots of the filtered receiver function, autocorrelation, and the resonance filter
Example
>>> # Initialize the plotfiltrf module: >>> from rfsed.ReverbFilter import plotfiltrf >>> # Define all the necessary parameters >>> # The FilteredRF data is a dictionary from the ResonanceFilt function >>> from rfsed.ReverbFilter import ResonanceFilt >>> FilteredRF = ResonanceFilt(rfstream, preonset) >>> savepath = 'path/to/saveplots' >>> format = 'jpg' >>> # Call the plotfiltrf function >>> plotfiltrf(FilteredRF, savepath, format)