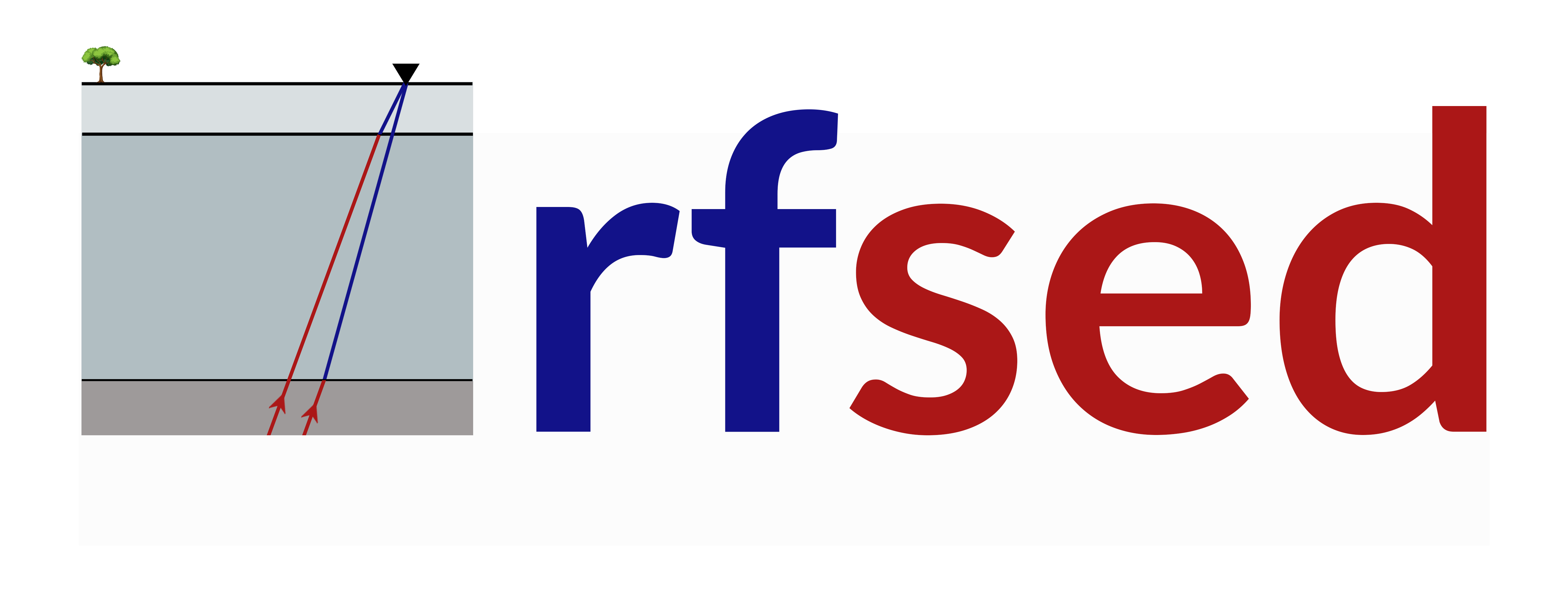
Module hkSeqYeck
- rfsed.hkSeqYeck.getamp(rfdata, tarray, t)
Get the amplitude of the receiver function at a specific time
- Parameters:
rfdata (numpy.ndarray) – receiver function data
tarray (numpy.ndarray) – time array
t (float) – time to get the amplitude
- Returns:
Amplitude of the receiver function at time t
- rfsed.hkSeqYeck.hkSeq(rfstreamSed, rfstreamMoho, preonset, HSed, KSed, VpSed, w1Sed, w2Sed, w3Sed, HMoho, KMoho, VpMoho, w1Moho, w2Moho, w3Moho, g=[75.0, 10.0, 15.0, 2.5], stack=None)
Sequential H-K stacking method for receiver function analysis after Yeck et al., 2013
- Parameters:
rfstreamSed (RFStream) – Stream object of receiver function for sediment layer (high frequency)
rfstreamMoho (RFStream) – Stream object of receiver function for Moho layer (lower frequency)
preonset (integer) – time in seconds before the P-arrival
HSed (numpy.ndarray) – Depth range for sediment layer
KSed (numpy.ndarray) – Vp/Vs range for sediment layer
VpSed (float) – Vp for sediment layer
w1Sed – Weight for Ps arrival
w2Sed – Weight for PpPs arrival
w3Sed – Weight for PsPs arrival
HMoho (numpy.ndarray) – Depth range for Moho layer
KMoho (numpy.ndarray) – Vp/Vs range for Moho layer
VpMoho (float) – Vp for Moho layer
w1Moho – Weight for Ps arrival
w2Moho – Weight for PpPs arrival
w3Moho – Weight for PpSs+PsPs arrival
g (list) – Gain for plotting
rmneg (bool) – Remove negative values in the stacking results
stack (bool) – Stack the receiver functions traces into one before hk stacking
- Returns:
Dictionary of results from the Sequential H-K stacking method
- Return type:
SequentialHKResult
Example
>>> # Initialize the hkSeq module: >>> from rfsed.hkSeqYeck import hkSeq >>> # Define input data and all the necessary parameters. The input data should >>> # be in the form of RFStream object from rf package >>> import numpy as np >>> from rf.rfstream import read_rf >>> rfstreamSed = read_rf('path/to/SedimentRF') >>> rfstreamMoho = read_rf('path/to/MohoRF') >>> preonset = 10 >>> HSed = np.linspace(0,10,201) >>> KSed = np.linspace(1.65,2.25,201) >>> VpSed = 3.0 >>> w1Sed, w2Sed, w3sed = [0.6, 0.3, 0.1] >>> HMoho = np.linspace(20,60,201) >>> KMoho = np.linspace(1.65,1.95,121) >>> VpMoho = 6.9 >>> w1Moho, w2Moho, w3Moho = [0.6, 0.3, 0.1] >>> g = [75.,10., 15., 2.5] >>> stack = False >>> # Call the hkSeq function >>> hkSeq(rfstreamSed, rfstreamMoho, preonset, HSed, KSed, VpSed, w1Sed, w2Sed, w3Sed, HMoho, KMoho, VpMoho, w1Moho, w2Moho, w3Moho, g, stack)
- rfsed.hkSeqYeck.plotSeqhk(SequentialHKResult, savepath, g=[75.0, 10.0, 15.0, 2.5], rmneg=True, format='jpg')
Plot the results from the Sequential H-K stacking method
- Parameters:
SequentialHKResult (dict) – Dictionary of results from the Sequential H-K stacking method (function: hkSequential)
g (list) – Gain for plotting
rmneg (bool) – Remove negative values in the stacking results
format (str) – Format of the figure
savepath (str) – Path to save the figure
- Returns:
Plot of the results from the Sequential H-K stacking method
Example
>>> # Initialize the plotSeqhk module: >>> from rfsed.hkSeqYeck import plotSeqhk >>> # Define input data (which is the result from the hkSeq function) >>> # and other plotting parameters. >>> SequentialHKResult = hkSeq(rfstreamSed, rfstreamMoho, preonset, HSed, KSed, VpSed, w1Sed, w2Sed, w3Sed, HMoho, KMoho, VpMoho, w1Moho, w2Moho, w3Moho, g, stack) >>> savepath = 'path/to/save/figure' >>> g = [75.,10., 15., 2.5] >>> rmneg = True >>> format = 'pdf' >>> # Call the plotSeqhk function >>> plotSeqhk(SequentialHKResult, savepath, g, rmneg, format)