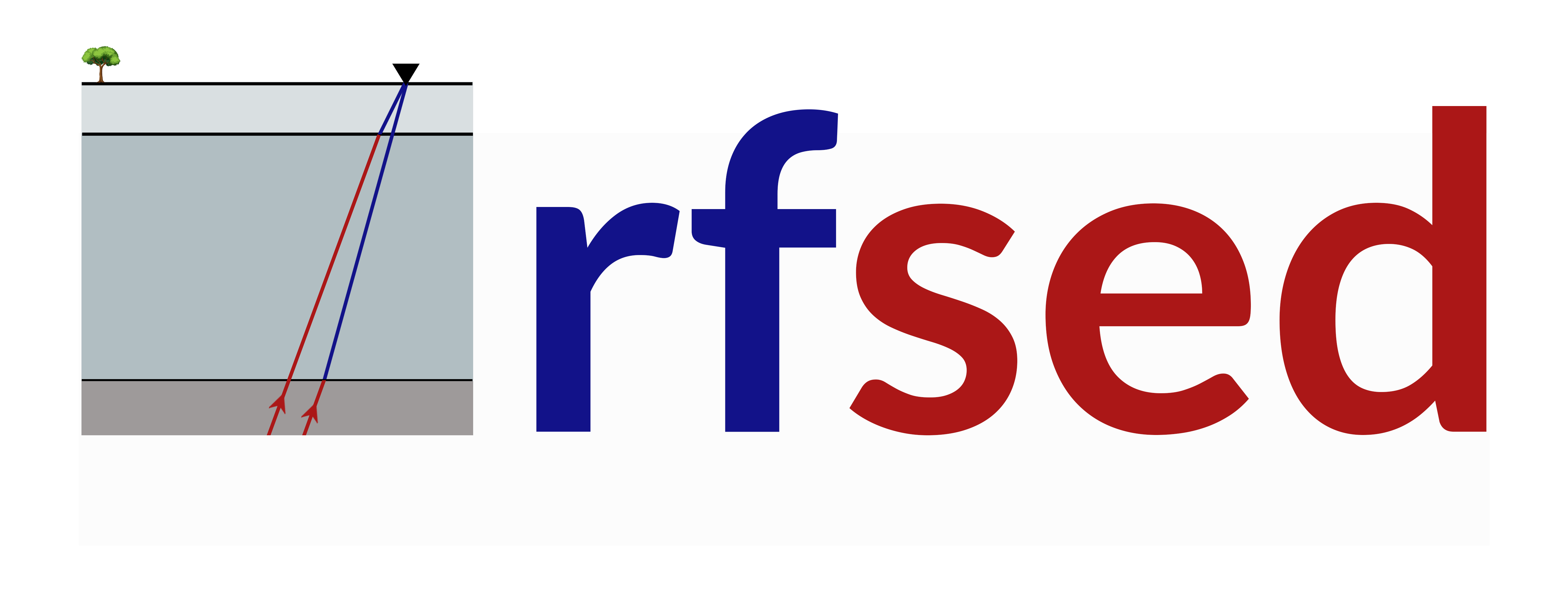
Module hkYu
- rfsed.hkYu.getamp(rfdata, tarray, t)
Get the amplitude of the receiver function at a specific time
- Parameters:
rfdata (numpy array) – receiver function data
tarray (numpy array) – time array
t (float) – time to get the amplitude
- Returns:
Amplitude of the receiver function at time t
- rfsed.hkYu.hkYu(FltResult, rayp, HSubSed, KSubSed, VpMoho, VpSed, VsSed, w1SubSed, w2SubSed, w3SubSed)
Modified H-K stacking method of Yu et al. (2015)
- Parameters:
FltResult (dict) – Dictionary of results from the resonance filtering method (function: Resonance_Filt)
rayp (float) – Ray parameter
HSubSed (numpy array) – Subsediment layer thickness array
KSubSed (numpy array) – Subsediment layer Vp/Vs array
VpMoho (float) – Moho Vp
VpSed (float) – Sediment Vp
w1SubSed (float) – Weight for the Subsediment Ps arrival at adjusted arrival time
w2SubSed (float) – Weight for the Subsediment Ppps arrival at adjusted arrival time
w3SubSed (float) – Weight for the Subsediment Psps+PpSs arrival at adjusted arrival time
- Returns:
Dictionary of results from the mofified H-K stacking method
- Return type:
HKYuResult
Example
>>> # Initialize the Modified H-K stacking method: >>> from rfsed.hkYu import hkYu >>> import numpy as np >>> # Define all the necessary parameters >>> # The FltResult are results from the resonance filtering method >>> # (see ReverbFilter module) >>> FltResult = {'filteredrf': filteredrf, 'time':time, 'tlag':tlag} >>> rayp = 0.04 >>> HSubSed = np.linspace(20,60,201) >>> KSubSed = np.linspace(1.65,1.95,121) >>> VpMoho = 6.9 >>> VpSed = 2.5 >>> VsSed = 1.4 >>> w1SubSed, w2SubSed, w3SubSed = [0.6, 0.3, 0.2] >>> # Call the hkYu function >>> hkYuResult = hkYu(FltResult, rayp, HSubSed, KSubSed, VpMoho, VpSed, VsSed, w1SubSed, w2SubSed, w3SubSed)
- rfsed.hkYu.plothkYu(hkYuResult, savepath, g=[75.0, 10.0, 15.0, 2.5], rmneg=True, format='jpg')
Plot the results of the Modified H-K stacking method of Yu et al. (2015)
- Parameters:
hkYuResult (dict) – Dictionary of results from the Modified H-K stacking method (function: hkYu)
savepath (str) – path to save the plots
g (list) – gain values for the plot (default: [75.,10., 15., 2.5])
rmneg (bool) – remove the stack values that is lower than zero (default: False)
format (str) – format of the plot (default: jpg)
- Returns:
Plot of the results from the Modified H-K stacking method
Example
>>> # Initialize the Modified H-K stacking plotting method: >>> from rfsed.hkYu import plothkYu >>> # Define all the necessary parameters >>> # The hkYuResult are results from the Modified H-K stacking >>> # method (see hkYu function) >>> hkYuResult = hkYu(FltResult, rayp, HSubSed, KSubSed, HSed, KSed, VpMoho, VpSed, VsSed, w1SubSed, w2SubSed, w3SubSed) >>> savepath = 'path/to/saveplots' >>> g = [75.,10., 15., 2.5] >>> rmneg = True >>> format = 'jpg' >>> # Call the plothkYu function >>> plothkYu(hkYuResult, savepath, g, rmneg, format)