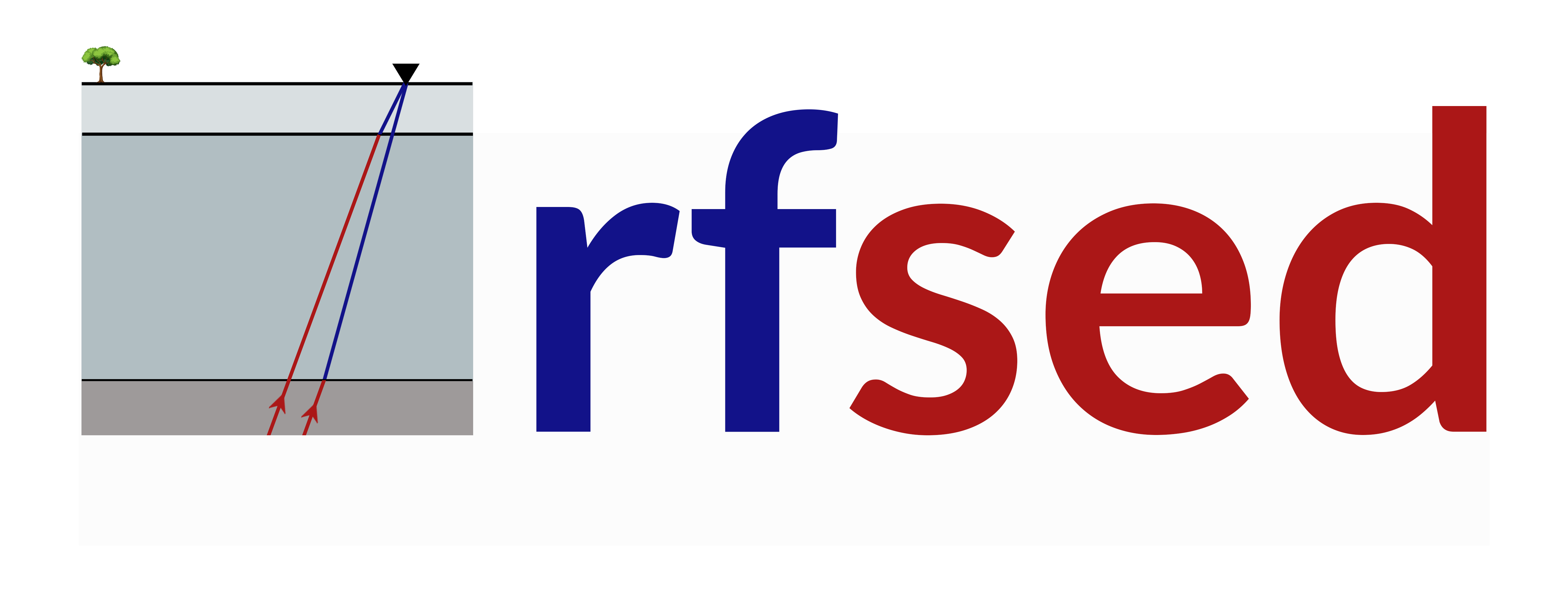
Module ExtractEqMultiproc
- rfsed.ExtractEqMultiproc.Add_sta_time_2cat(catalogfile, stalat, stalon, Request_window)
Add station latitude, longitude and request time window to each event in the catalog
- Parameters:
catalogfile (str) – Path to the catalog file
stalat (float) – Station latitude
stalon (float) – Station longitude
Request_window (list [start, end]) – Request time window relative to first P arrival (time before P arrival, time after P arrival)
- Returns:
Catalog of events with station latitude, longitude and request time window added to each event
Example
>>> # Initialize the Add_sta_time_2cat function: >>> from rfsed.ExtractEqMultiproc import Add_sta_time_2cat >>> # Define the input parameters: >>> catalogfile = 'path/to/catalogfile' >>> stalat = 52.0 >>> stalon = 4.0 >>> Request_window = [-50, 150] >>> # Add the station latitude, longitude and request time window to the >>> # catalog using the Add_sta_time_2cat function: >>> catalog = Add_sta_time_2cat(catalogfile, stalat, stalon, Request_window)
- rfsed.ExtractEqMultiproc.Eq_times(catalog)
Get the earthquake start and end times in obspy UTCDateTime for the requested time window relative to the first P arrival for each event in the catalog. The theoretical P wave arrival time is calculated using the IASP91 reference model
- Parameters:
catalog (obspy.core.event.catalog.Catalog) – Catalog with station latitude, longitude and request time window (output of Add_cat_sta_time)
- Returns:
Dictionary of Earthquake start and end time for the requested time window relative to the first P arrival
Example
>>> # Initialize the Eq_times function: >>> from rfsed.ExtractEqMultiproc import Eq_times >>> # Define the input parameters (output from the Add_cat_sta_time function): >>> catalog = Add_cat_sta_time(catalogfile, stalat, stalon, Request_window) >>> # Get the earthquake start and end times for the requested time window >>> # relative to the first P arrival using the Eq_times function: >>> timewindow = Eq_times(catalog)
- rfsed.ExtractEqMultiproc.Get_Eqtimes_multiproc(catalog, nproc)
Run the Eq_times function in parallel for all events in the catalog
- Parameters:
catalog (obspy.core.event.catalog.Catalog) – Catalog with station latitude, longitude and request time window (output of Add_cat_sta_time)
nproc (int) – Number of processors to use
- Returns:
List of dictionaries of Earthquake start and end times (in obspy UTCDateTime) for the requested time window relative to the first P arrival
Example
>>> # Initialize the Get_Eqtimes_multiproc function: >>> from rfsed.ExtractEqMultiproc import Get_Eqtimes_multiproc >>> # Define the input parameters catalog and nproc (number of processors to use) >>> # he catalog is an output from the Add_cat_sta_time function >>> catalog = Add_cat_sta_time(catalogfile, stalat, stalon, Request_window) >>> nproc = 4 >>> # Get the earthquake start and end times for the requested time window >>> # relative to the first P arrival using the Get_Eqtimes_multiproc function: >>> timewindow = Get_Eqtimes_multiproc(catalog, nproc)
- rfsed.ExtractEqMultiproc.Get_EqStream_multiproc(inputparams)
Extract the earthquake waveform data from the local data files for the requested time window relative to the first P arrival
- Parameters:
inputparams (list) – List of input parameters (datafiles, timewindow)
- Returns:
Stream of earthquake data for the requested time window relative to the first P arrival
Example
>>> # Initialize the Get_EqStream_multiproc function: >>> from rfsed.ExtractEqMultiproc import Get_EqStream_multiproc >>> # Define the input parameters datafiles and timewindow >>> datafiles = ['path/to/datafiles'] >>> timewindow = [{'eqstart':eqstart, 'eqend':eqend, 'onset':onset}] >>> inputparams = (datafiles, timewindow) >>> # Get the earthquake waveform data for the requested time window relative >>> # to the first P arrival using the Get_EqStream_multiproc function: >>> Datast = Get_EqStream_multiproc(inputparams)
- rfsed.ExtractEqMultiproc.ExtractEq_Multiproc(datafiles, timewindow, nproc, filename)
Runs the Get_EqStream_multiproc function in parallel for each data file, extracts the earthquake data for the requested time window relative to the first P arrival and save the data to a file
- Parameters:
datafiles (list) – List of data files
timewindow (dict) – Dictionary of Earthquake start and end time for the requested time window relative to the first P arrival
nproc (int) – Number of processors to use
filename (str) – Path to save the extracted earthquake data
- Returns:
Extracted earthquake data to the specified file path
Example
>>> # Initialize the ExtractEq_Multiproc function: >>> from rfsed.ExtractEqMultiproc import ExtractEq_Multiproc >>> # Define the input parameters datafiles, timewindow, nproc and filename >>> datafiles = ['path/to/datafiles'] >>> # time window is an output from the Eq_times or Get_Eqtimes_multiproc >>> # functions >>> timewindow = [{'eqstart':eqstart, 'eqend':eqend, 'onset':onset}] >>> nproc = 4 >>> filename = 'path/to/newfile' >>> # Extract the earthquake waveform data for the requested time window relative >>> # to the first P arrival using the ExtractEq_Multiproc function: >>> ExtractEq_Multiproc(datafiles, timewindow, nproc, filename)