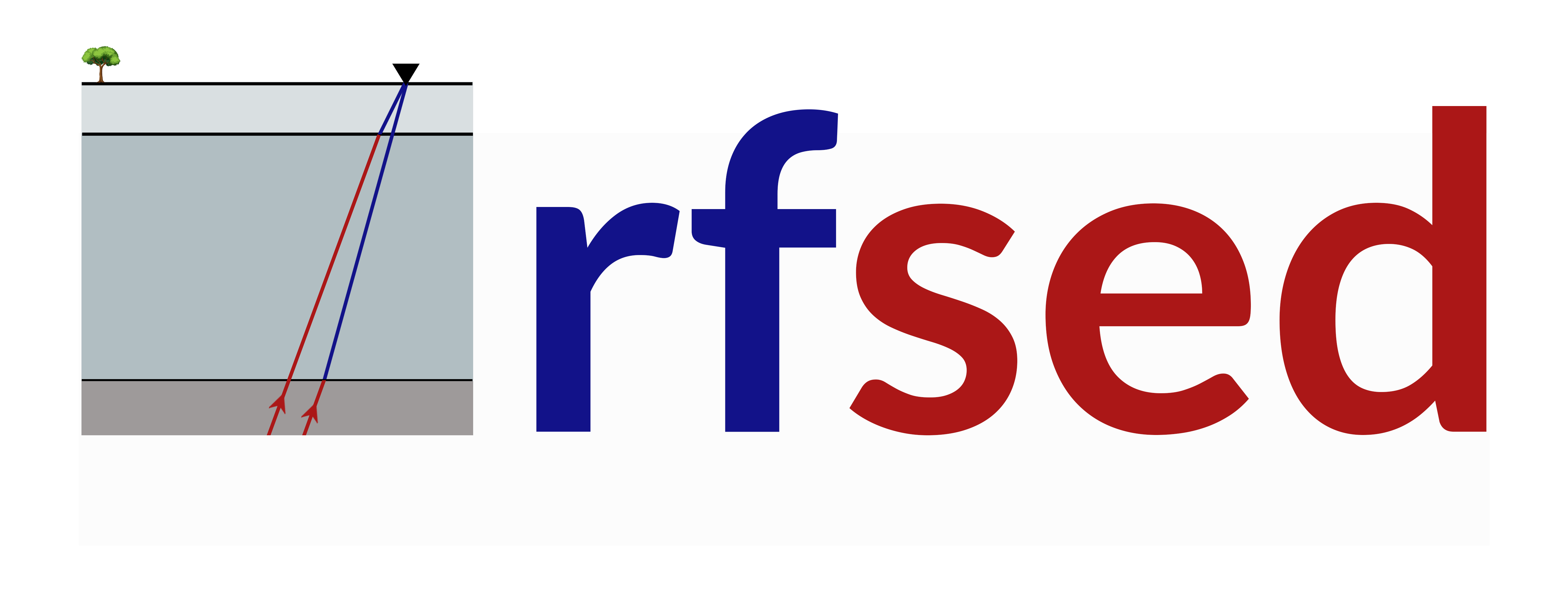
Module WaveformFittingMultiproc
- rfsed.WaveformFittingMultiproc.WaveformPara(rfstream, preonset, HSed, VpSed, VpCrust, rayp, KMoho, HMoho, gaussian)
Calculate the Model Parameters for the Waveform Fitting
- Parameters:
rfstream (RFStream) – RFStream object containing the receiver functions
preonset (integer) – time in seconds before the P-arrival
HSed (float) – Sediment Layer Thickness
VpSed (float) – P-wave velocity in the Sediment
VpCrust (float) – P-wave velocity in the Crust
rayp (float) – Ray Parameter (s/km)
KMoho (numpy array) – numpy array of Vp/Vs ratios for the Moho
HMoho (numpy array) – numpy array of Moho depths
gaussian (float) – Gaussian Parameter used in the receiver function calculation (e.g. 1.25)
- Returns:
Dictionary of Model Parameters
Example
>>> # Initialize the WaveformPara module: >>> from rfsed.WaveformFittingMultiproc import WaveformPara >>> import numpy as np >>> # Define all the necessary parameters >>> # rfstream is a RFStream object containing the receiver functions (based on rf) >>> rfstream = rfstream >>> preonset = 10 >>> HSed = 0.8 >>> VpSed = 2.5 >>> VpCrust = 6.5 >>> rayp = 0.06 >>> KMoho = np.arange(1.65, 1.95, 201) >>> HMoho = np.arange(20, 50, 201) >>> gaussian = 1.25 >>> # Call the WaveformPara function >>> ModelParams = WaveformPara(rfstream, preonset, HSed, VpSed, VpCrust, rayp, KMoho, HMoho, gaussian)
- rfsed.WaveformFittingMultiproc.WaveformFit_multiproc(inputparams)
Calculate the Waveform Fitting for each Moho depth
- Parameters:
inputparams (list) – List of input parameters (HMoho, OtherParams)
- Returns:
List of Waveform Fitting Results
- rfsed.WaveformFittingMultiproc.run_waveformfitting(nproc, HMoho, ModelParams)
Run the Waveform Fitting Function in parallel for each Moho depth
- Parameters:
nproc (int) – Number of processors to use
HMoho (numpy array) – numpy array of Moho depths
ModelParams (dict) – Dictionary of Model Parameters from function WaveformPara
- Returns:
List of Waveform Fitting Results
Example
>>> # Initialize the run_waveformfitting module: >>> from rfsed.WaveformFittingMultiproc import run_waveformfitting >>> # Define all the necessary parameters >>> import numpy as np >>> nproc = 20 >>> HMoho = np.arange(20, 50, 201) >>> # ModelParams is a dictionary of model parameters which is an output from the >>> # WaveformPara function (see WaveformPara function for more details) >>> ModelParams = WaveformPara(rfstream, preonset, HSed, VpSed, VpCrust, rayp, KMoho, HMoho, gaussian) >>> # Call the run_waveformfitting function >>> WaveformFitResults = run_waveformfitting(nproc, HMoho, ModelParams)
- rfsed.WaveformFittingMultiproc.plotbestmodel(WaveformResults, ModelParams, wtCorr, wtRMSE, wtPG, savepath, format)
Plot the best model from the Waveform Fitting Results
- Parameters:
WaveformResults (list) – List of Waveform Fitting Results
ModelParams (dict) – Dictionary of Model Parameters
wtCorr (float) – Weighting for the Correlation Coefficient
wtRMSE (float) – Weighting for the Root-mean-square Error
wtPG (float) – Weighting for the Phase Goodness of Fit
savepath (str) – Path to save the plot
format (str) – Format to save the plot
- Returns:
Plot of the best model from the Waveform Fitting Results
Example
>>> # Initialize the plotbestmodel module: >>> from rfsed.WaveformFittingMultiproc import plotbestmodel >>> # Define all the necessary parameters >>> # WaveformResults is a list of Waveform Fitting Results, >>> # which is an output of the run_waveformfitting function >>> # (see run_waveformfitting function for more details) >>> WaveformResults = run_waveformfitting(nproc, HMoho, ModelParams) >>> # ModelParams is a dictionary of model parameters which is an output from >>> # the WaveformPara function (see WaveformPara function for more details) >>> ModelParams = WaveformPara(rfstream, preonset, HSed, VpSed, VpCrust, rayp, KMoho, HMoho, gaussian) >>> wtCorr, wtRMSE, wtPG = [0.4, 0.4, 0.2] >>> savepath = 'path/to/save/plot' >>> format = 'png' >>> # Call the plotbestmodel function >>> plotbestmodel(WaveformResults, ModelParams, wtCorr, wtRMSE, wtPG, savepath, format)